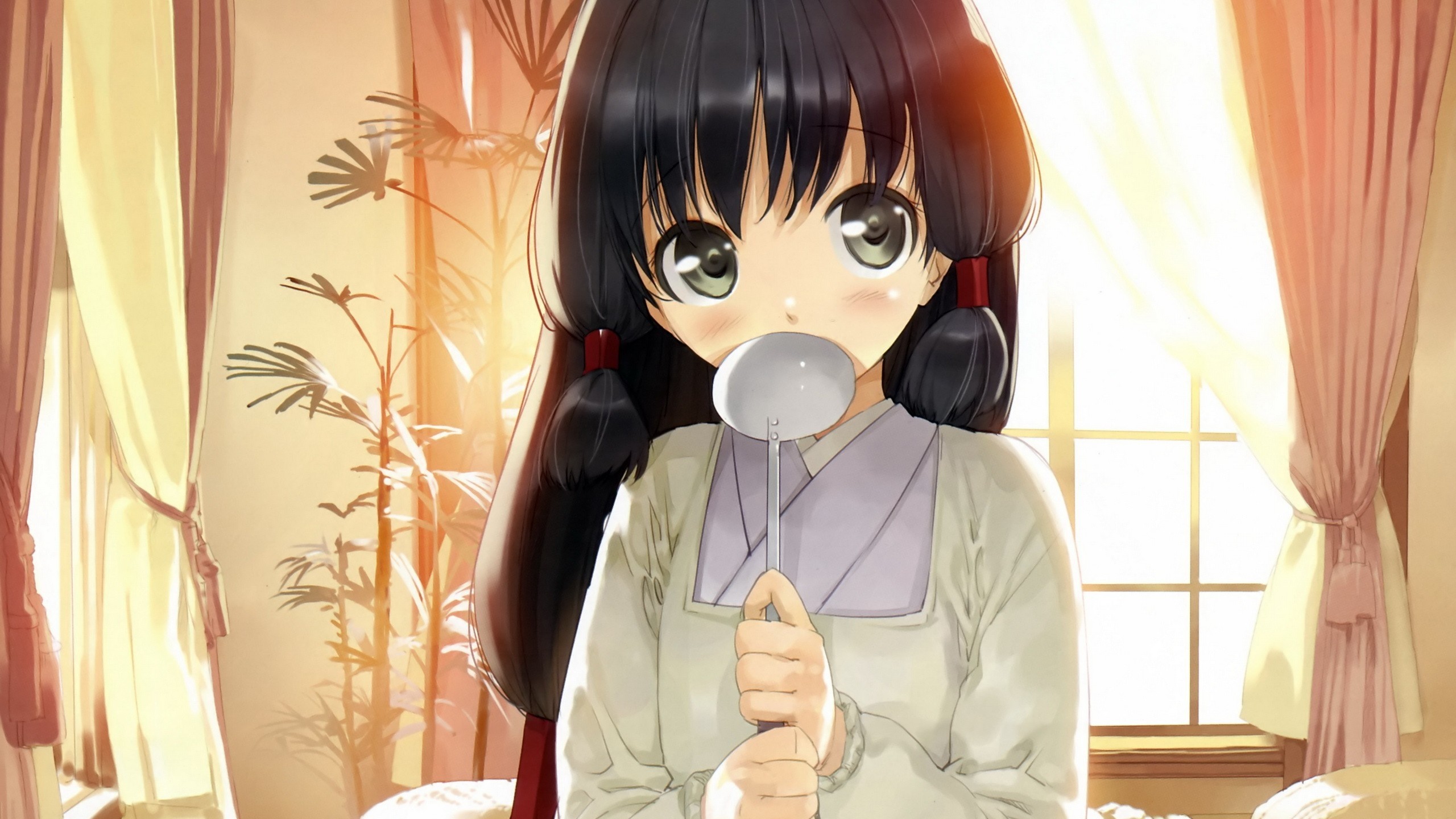
一、导入依赖
在 pom.xml
文件中添加依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
二、配置邮件服务信息
在 aplication.xml
中写入如下配置:
Spring:
mail:
default-encoding: UTF-8
protocol: smtp
host: smtp.yeah.net # 选择的邮箱类别,此处为网易yeah.net邮箱
username: xxxx@yeah.com # 您的邮箱
password: xxxxxx # 您的 smtp 登录密钥
常用邮箱类别及对应的host如下表
邮箱 | 格式 | host |
---|---|---|
xxx@qq.com | smtp.qq.com | |
yeah | xxx@yeah.net | smtp.yeah.net |
网易163 | xxx@163.com | smtp.163.com |
新浪 | xxx@sina.com.cn | smtp.sina.com.cn |
gmail | xxx@gmail.com | smtp.gmail.com |
三、编写邮箱服务类
package com.xxx.service;
import com.xxx.myblog.service.EmailService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
import java.util.Date;
@Slf4j
@Service
public class EmailService{
@Autowired
private JavaMailSender javaMailSender;
@Value("${spring.mail.username}")
private String from;
//发给别人
@Async("taskExecutor") //异步发送
public void sendSimpleEmail(String toEmail, String title, String content) {
SimpleMailMessage message = new SimpleMailMessage();
message.setSubject(title);
// 从哪里发送
message.setFrom(from);
// 到哪里 可设置多个,实现群发
message.setTo(toEmail);
//发送日期
message.setSentDate(new Date());
//发送内容
message.setText(content);
try {
javaMailSender.send(message);
log.info("邮件发送成功");
} catch (Exception e) {
log.error("邮件发送失败");
}
}
}
四、设置异步发送
4.1 先开启异步
在 Aplication.java
中添加注解
package com.xxx;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableAsync;
@EnableAsync //添加注解
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
4.2 添加异步配置类
package com.xxx.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.task.TaskExecutor;
import org.springframework.scheduling.annotation.EnableAsync;
import org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor;
import java.util.concurrent.ThreadPoolExecutor;
@Configuration
@EnableAsync // 开启异步配置
public class AsyncTaskConfig {
@Bean
public TaskExecutor taskExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
//设置核心线程数
executor.setCorePoolSize(10);
//设置最大线程数
executor.setMaxPoolSize(20);
//缓冲队列200:用来缓冲执行任务的队列
executor.setQueueCapacity(200);
//线程活路时间 60 秒
executor.setKeepAliveSeconds(60);
//线程池名的前缀:设置好了之后可以方便我们定位处理任务所在的线程池
executor.setThreadNamePrefix("task-");
//设置拒绝策略
executor.setRejectedExecutionHandler(new ThreadPoolExecutor.CallerRunsPolicy());
executor.setWaitForTasksToCompleteOnShutdown(true);
return executor;
}
}
五、使用
直接调用即可
@SpringBootTest
class ApplicationTests {
@Autowired
private EmailService emailService;
@Test
void contextLoads() {
emailService.sendSimpleEmail("xxx@qq.com", "测试", "Hello Wolrd!");
}
}
标题: | SpringBoot整合邮件发送 |
---|---|
链接: | https://www.fightingok.cn/detail/146 |
更新: | 2022-09-18 22:42:51 |
版权: | 本文采用 CC BY-NC-SA 3.0 CN 协议进行许可 |